Emotion Styled Component Cascading 문제
Emotion에서 Styled Component 를 사용하면 해당 컴포넌트의 클래스 이름이 재정의된다.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| const StyledTabPanel = styled.div<TabPanelProps<"div">>` padding: 5px; border: 2px solid hsl(219deg 1% 72%); border-radius: 0 5px 5px; background: hsl(220deg 43% 99%); min-height: 10em; min-width: 550px; overflow: auto; display: ${(props) => props.dataIndex === +(props.id?.slice(-1) as unknown as number) ? "block" : "none"}; `;
function TabPanel({ children, className, ...restProps }: TabPanelProps<"div">) { const { selected, setSelected } = useTabsContext();
return ( <StyledTabPanel role="tabpanel" tabIndex={0} dataIndex={selected} className={className} {...restProps} > {children} </StyledTabPanel> ); }
|
styled component를 사용하여 TabPanel 컴포넌트를 생성하였다. 브라우저에서 해당 요소를 확인해보면 다음과 같이 알 수 없는 클래스로 나오는 것을 알 수 있다.
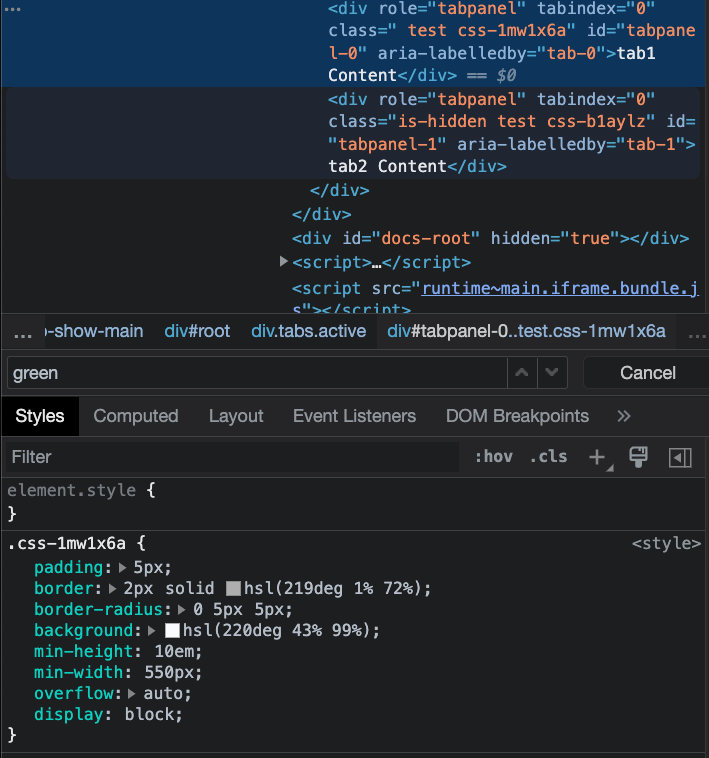
TypeScript 오류 - 사용량 덮어씜
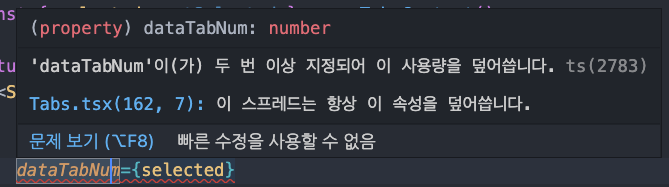
props를 생성하고 해당 props를 통해 styled Component에서 조건부 스타일을 주려고 한다.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| type Component<T extends React.ElementType> = { className?: string; children?: React.ReactNode; } & React.ComponentPropsWithRef<T>;
type TabPanelProps<T extends React.ElementType> = { dataIndex?: TabsContextValue["selected"]; } & Component<T>;
const StyledTabPanel = styled.div<TabPanelProps<"div">>` padding: 5px; border: 2px solid hsl(219deg 1% 72%); border-radius: 0 5px 5px; background: hsl(220deg 43% 99%); min-height: 10em; min-width: 550px; overflow: auto; display: ${(props) => props.dataIndex === +(props.id?.slice(-1) as unknown as number) ? "block" : "none"}; `;
function TabPanel({ children, className, ...restProps }: TabPanelProps<"div">) { const { selected, setSelected } = useTabsContext();
return ( <StyledTabPanel role="tabpanel" tabIndex={0} dataIndex={selected} className={className} {...restProps} > {children} </StyledTabPanel> ); }
|
- 해당 타입을 정의할 때, 필수가 아닌 선택 사항으로 정의해주어서 해결하였다.
- styled component 타입 지정 시 해당 컴포넌트의 props라고 지정을 해줘야지만 props에서 찾을 수 있다.
댓글 공유